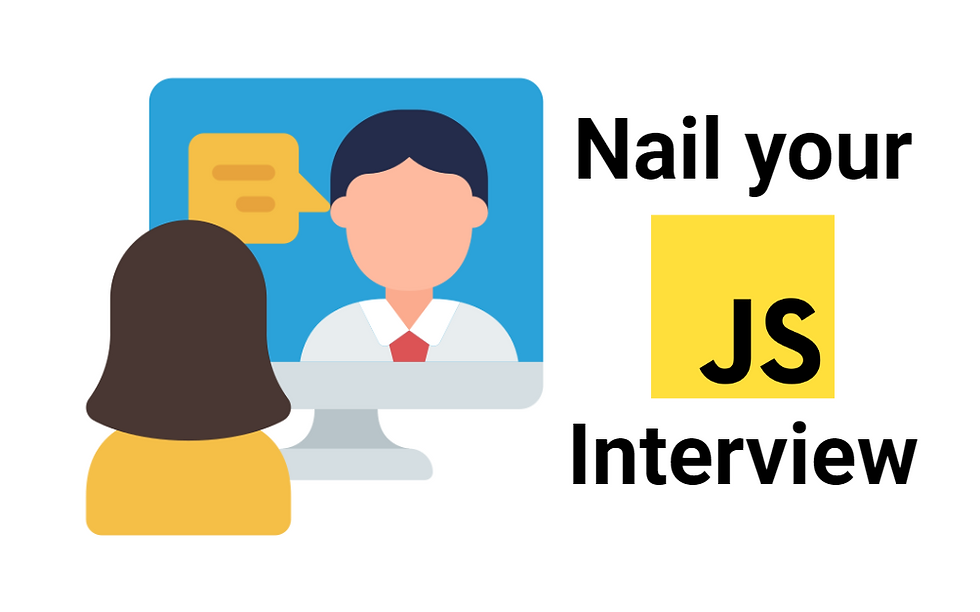
JavaScript is essential for modern web development, enabling dynamic and interactive experiences online. Mastering it is crucial for developers at all levels, from enhancing user interfaces to driving server-side applications. In today's tech landscape, proficiency in JavaScript is a must for staying competitive and driving innovation.
Want to ace JavaScript and prepare for your interview but don't know where to start?
No worries, in this post, I will outline the most commonly asked JavaScript questions by interviewers and provide comprehensive answers to each.
They are divided into three parts:
Beginner Questions
Q-BE1. What is JavaScript?
JavaScript is lightweight, interpreted programming language commonly used in web development to make websites more interactive and engaging. It is a very powerful client-side scripting language. JavaScript is used mainly for enhancing the interaction of a user with the webpage. In other words, you can make your webpage more lively and interactive, with the help of JavaScript. JavaScript is also being used widely in game development and Mobile application development.
Q-BE2. What is the differences between Java and JavaScript?
Aspect | Java | JavaScript |
Type | Object-oriented programming language | Lightweight, interpreted scripting language |
Purpose | General-purpose (standalone apps, enterprise systems, Android apps) | Primarily web development (interactive websites) and server-side development (Node.js) |
Execution Environment | Java Virtual Machine (JVM) | Web browsers, Node.js, other environments |
Syntax | Verbose, similar to C++, strongly typed | Flexible, less verbose, loosely typed |
Compilation/Interpretation | Compiled to bytecode, executed by JVM | Interpreted, executed by JavaScript engine |
Concurrency | Supports multithreading | Event-driven, non-blocking I/O, single-threaded with event loop |
Use Cases | Desktop applications, enterprise applications, Android apps | Client-side web development, enhancing UI, server-side (Node.js), mobile apps, games |
Learning Curve | Steeper learning curve | Easier for beginners, especially in web development |
Q-BE3. What are the data types supported by JavaScript?
Primitive types - immutable values:
Type | Usage and typeof return | Object wrapper |
Boolean | Represents a logical entity and is inhabited by true or false. typeof returns "boolean" | Boolean |
Number | Double precision 64-bit binary format. MAX_VALUE is 2^1024 and MIN_VALUE is 2^-1074 but can only safely store ^53 by MAX_SAFE or MIN_SAFE. Outside of these will be automatically converted. typeof returns "number" | Number |
BigInt | Can represent integers with arbitrary magnitude. Can safely store and operate on large integers beyond Number.MAX_SAFE. typeof returns "bigint" | BigInt |
String | Represents textual data, and it is immutable, once created, it is not possible to modify. While representing complex data, it is advised to parse strings and use appropriate abstraction. typeof returns "string" | String |
Symbol | Is unique and immutable primitive that may be used as the key of an Object property. The purpose is to create unique property keys that not clash with keys form other code. typeof returns "symbol" | Symbol |
Null | Is inhabited by one value: null typeof returns "object" | N/A |
Undefined | Is inhabited by one value: undefined. Conceptually, undefined indicates the absence of a value, while null indicates the absence of an object. typeof returns "undefined" | N/A |
Mutable value - Objects:
Object is a value in memory which is possibly referenced by an identifier. In JavaScript, objects are the only mutable value.
Q-BE4. What are features of JavaScript
They are:
Lightweight and Interpreted: JavaScript is executed line-by-line in the web browser, which makes it lightweight and fast.
Dynamic Typing: Variables in JavaScript do not require a type declaration and can hold different data types at different times.
First-Class Functions: Functions in JavaScript are treated as first-class objects, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
Event-Driven: JavaScript allows for the creation and handling of events, making it ideal for creating interactive web pages.
Asynchronous Programming: Supports asynchronous operations through callbacks, promises, and async/await, enabling non-blocking code execution.
Prototype-Based Inheritance: Uses prototypes for inheritance rather than classical object-oriented inheritance, providing a flexible and dynamic way of sharing properties and methods.
Client-Side Scripting: Runs primarily on the client side (in the web browser), allowing for dynamic content updates without refreshing the page.
Cross-Platform Compatibility: Works on all major web browsers and platforms, ensuring wide accessibility.
Standardized: ECMAScript standardizes JavaScript, ensuring consistency across different implementations and platforms.
Rich Ecosystem: Extensive libraries and frameworks (like React, Angular, and Vue.js) enhance development capabilities and efficiency.
Q-BE5. Is JavaScript a case-sensitive language?
Yes, JavaScript is a case-sensitive language. This means that it distinguishes between uppercase and lowercase characters. For example, the variables myVariable and MyVariable would be considered distinct and separate entities in JavaScript. Similarly, keywords, function names, and other identifiers must be consistently cased correctly.
Q-BE6. Advantages of JavaScript?
Following are the advantages of using JavaScript:
Speed: JavaScript is an “interpreted” language, so it cuts down on the time needed for compilation in other languages like Java.
Less server interaction: The language runs on the client side rather than the server. Thus, the server doesn’t have to deal with the stress of executing JavaScript. Once this burden is reduced, the server will function more quickly and concentrate on other tasks like data management.
Rich interface: JavaScript offers developers a variety of interfaces to build engaging websites. Websites with drag-and-drop elements or sliders may have a more robust user experience. This increases user interaction with the website.
Interoperability: JavaScript seamlessly integrates with other programming languages, so many developers favor using it to create various apps. Any webpage or script of another computer language can incorporate it.
Ease of Use: JavaScript is one of the simplest languages to learn, particularly for web programming. It has been designed to be simple for web developers to understand and use.
Popularity with Powerful Frameworks: JavaScript is one of the most popular languages for web development. It is an important factor for every working website. Many commanding framework display ready-to-use codes. Such codes are all easy to comprehend and troubleshoot.
Q-BE7. Which is faster between JavaScript and an ASP script?
JavaScript is faster. JavaScript is a client-side language, and thus it does not need the assistance of the webserver to execute. On the other hand, ASP is a server-side language and hence is always slower than JavaScript. It now is also a server-side language (Nodejs).
Q-BE8. How to create an object in JavaScript
We can create object using object literal as below:
let Car = {
brand: "BMW",
series: "M4"
}
Q-BE9. What is anonymous function?
An anonymous function is a function without having a name. It is widely used for short, one-off tasks where naming the function is not necessary.
An anonymous function is not accessible after its initial creation, it can only be accessed by a variable it is stored in as a function as a value. An anonymous function can also have multiple arguments, but only one expression.
var anonymous = function() {
alert('This is anonymous function');
};
anon();
Q-BE10. How to create Array in JavaScript?
Use array literal:
let a = [];
let b = ["apple", "banana", "orange"];
Q-BE11. What is name function and how to define it?
A named function in JavaScript is a function that has a name specified when it is defined. Named functions are useful for code readability, debugging, and can also be referenced recursively within their own body.
function functionName() {
// function body
}
Benefits of named functions:
Readability: Named functions make your code easier to understand and maintain.
Debugging: When an error occurs, the stack trace will include the name of the function, making it easier to identify where the error happened.
Recursion: Named functions can call themselves recursively by using their name within the function body.
Q-BE12. Can we assign an anonymous function to a variable and pass it as an argument to another function?
Yes, you can assign an anonymous function to a variable and pass it as an argument to another function in JavaScript. This is a common practice, especially in functional programming and when working with higher-order functions (functions that take other functions as arguments or return functions).
const greet = function(name) {
console.log("Hello, " + name + "!");
};
function executeFunction(fn, value) {
fn(value);
}
executeFunction(greet, "Charlie"); // Output: Hello, Charlie!
Here, the greet variable holds an anonymous function, which is then passed to executeFunction and called with the argument "Charlie".
These techniques are powerful tools in JavaScript, enabling you to write flexible and reusable code.
Q-BE13. What is argument objects? How to get the type of arguments passed to a function?
JavaScript variable arguments represents the arguments that are being passed to a function. Using typeof operator, the type of object can be returned.
function function(x){
console.log(typeof x, arguments.length);
}
function(); //==> "undefined", 0
function(3); //==> "number", 1
function("1", "2", "3"); //==> "string", 3
Q-BE14. Explain Scope and Scope Chain in JavaScript
Scope in JS determines the accessibility of variables and functions at various parts of one’s code.
There are three types of scopes in JS:
Global Scope
Local or Function Scope
Block Scope
Global Scope: Variables or functions declared in the global namespace have global scope, which means all the variables and functions having global scope can be accessed from anywhere inside the code.
var globalVariable = "Hello world";
function sendMessage(){
return globalVariable; // can access globalVariable since it's written in global space
}
function sendMessage2(){
return sendMessage(); // Can access sendMessage function since it's written in global space
}
sendMessage2(); // Returns "Hello world"
Function Scope: Any variables or functions declared inside a function have local/function scope, which means that all the variables and functions declared inside a function, can be accessed from within the function and not outside of it.
function scopeFunction(){
var a = 2;
var multiplyBy2 = function(){
console.log(a*2); // Can access variable "a" since a and multiplyBy2 both are written inside the same function
}
}
console.log(a); // Throws reference error since a is written in local scope and cannot be accessed outside
multiplyBy2(); // Throws reference error since multiplyBy2 is written in local scope
Block Scope: Block scope is related to the variables declared using let and const. Variables declared with var do not have block scope. Block scope tells us that any variable declared inside a block { }, can be accessed only inside that block and cannot be accessed outside of it.
{
let x = 45;
}
console.log(x); // Gives reference error since x cannot be accessed outside of the block
for(let i=0; i<2; i++){
// do something
}
console.log(i); // Gives reference error since i cannot be accessed outside of the for loop block
// NOTE
for(var y=0; y<2; y++){
// do something
}
console.log(y); // Return 2 as var do not have block scope
Scope Chain: JavaScript engine also uses Scope to find variables. Let’s understand that using an example:
var y = 24;
function favFunction(){
var x = 667;
var anotherFavFunction = function(){
console.log(x); // Does not find x inside anotherFavFunction, so looks for variable inside favFunction, outputs 667
}
var yetAnotherFavFunction = function(){
console.log(y); // Does not find y inside yetAnotherFavFunction, so looks for variable inside favFunction and does not find it, so looks for variable in global scope, finds it and outputs 24
}
anotherFavFunction();
yetAnotherFavFunction();
}
favFunction();
As you can see in the code above, if the JS engine does not find the variable in local scope, it tries to check for the variable in the outer scope. If the variable does not exist in the outer scope, it tries to find the variable in the global scope. If the variable is not found in the global space as well, a reference error is thrown.
More info: https://www.w3schools.com/js/js_scope.asp
Q-BE15. What is purpose of "this" keyword in JavaScript?
The "this" keyword refers to the object it belongs to (from where it was called). Depending on the context, this might have several meanings. This pertains to the global object in a function and the owner object in a method, respectively.
Example 1:
function doSomething() {
console.log(this);
}
doSomething();
// Since the function is invoked in the global context, the function is a property of the global object. Therefore, the output of the above code will be the global object. Since we ran the above code inside the browser, the global object is the window object.
Example 2:
var obj = {
name: "Batman",
getName: function(){
console.log(this.name);
}
}
var getName = obj.getName;
var obj2 = {name:"Superman", getName };
obj2.getName();
// Although the getName function is declared inside the object obj, at the time of invocation, getName() is a property of obj2, therefore the "this" keyword will refer to obj2. So output is "Superman".
Example 3:
var obj1 = {
address : "Paris, New York",
getAddress: function(){
console.log(this.address);
}
}
var getAddress = obj1.getAddress;
var obj2 = {name:"Superman"};
obj2.getAddress();
// The output will be an error. Although in the code above, this keyword refers to the object obj2, obj2 does not have the property "address", hence the getAddress function throws an error.
Q-BE16. How you can submit a form using JavaScript?
To submit a form using JavaScript use:
document.form[0].submit();
Q-BE17. How can the style/class of an element be changed?
It can be done in the following way:
document.getElementById("myText").style.fontSize = "14";
// or
document.getElementById("myText").className = "anyclass";
Q-BE18. How can a particular frame be targeted, from a hyperlink, in JavaScript?
This can be done by including the name of the required frame in the hyperlink using the ‘target’ attribute.
<a href="/newpage.htm" target="newframe">>New Page</a>
Q-BE19. What boolean operators can be used in JavaScript?
The "And" operator (&&), "Or" Operator (||), and the "Not" Operator (!) can be used in JavaScript.
*Operators are without the parenthesis.
Q-BE20. What is the distinction between client-side and server-side JavaScript?
Client-side JavaScript is made up of two parts, a fundamental language and predefined objects for performing JavaScript in a browser. JavaScript for the client is automatically included in the HTML pages. At runtime, the browser understands this script.
Server-side JavaScript, involves the execution of JavaScript code on a server in response to client requests. It handles these requests and delivers the relevant response to the client, which may include client-side JavaScript for subsequent execution within the browser.
Q-BE21. What is callback?
A callback is a function that will be executed after another function gets executed. In JS, functions are treated as first-class citizens, they can be used as an argument of another function, can be returned by another function, and can be used as a property of an object.
function divideByHalf(sum){
console.log(Math.floor(sum / 2));
}
function multiplyBy2(sum){
console.log(sum * 2);
}
function operationOnSum(num1,num2,operation){
var sum = num1 + num2;
operation(sum);
}
operationOnSum(3, 3, divideByHalf); // Outputs 3
operationOnSum(5, 5, multiplyBy2); // Outputs 20
Both divideByHalf and multiplyBy2 functions are used as callback functions in the code above.
These callback functions will be executed only after the function operationOnSum is executed.
Therefore, a callback is a function that will be executed after another function gets executed.
Q-BE22. Name some built-in methods and the values returned by each
Built-in method | Values |
charAt(x) | Returns the character at specified index. |
concat() | Joins two or more strings. |
indexOf() | Returns index of the first occurrence of the specified value. |
forEach() | Iterates through each elements in array. |
length() | Returns the length of the string. |
pop() | Removes the last element from an array and returns new length of array. |
push() | Adds one or more elements to the end of array and returns new length of array. |
reverse() | Reverses the order of the elements of array. |
shift() | Removes the first element from an array and returns that removed element. |
unshift() | Adds the specified elements to the beginning of an array and returns the new length of the array. |
Q-BE23. What are the variable naming conventions in JavaScript?
There are some rules we should comply:
JavaScript reserved keyword are not allowed to use in variable name. For example, "break" or "boolean" and so on.
Variable names cannot start with a number (0-9). They must begin with a letter or underscore character. For example, 123456name is invalid, but _123456name or name123456 is valid.
Variable names are case sensitive. For example, varName and varname are 2 different variables.
Q-BE24. What is the working of timers in JavaScript?
Timers are used to execute a piece of code at a set time or repeat the code in a given interval. This is done by using the functions setTimeout, setInterval, and clearInterval.
The setTimeout(function, delay) function is used to start a timer that calls a particular function after the mentioned delay.
The setInterval(function, delay) function repeatedly executes the given function in the mentioned delay and only halts when canceled.
The clearInterval(id) function instructs the timer to stop.
Timers are operated within a single thread, and thus events might queue up, waiting to be executed.
Q-BE25. Which symbol is used for comments in JavaScript?
// Single line comment
/* Multi
Line
Comment
*/
Q-BE26. What are JavaScript Cookies?
Cookies are the small test files stored in a computer, and they get created when the user visits the websites to store information that they need. Examples could be User Name details and shopping cart information from previous visits.
Q-BE27. How to create cookie using JavaScript?
The simplest way yo create a cookie is to assign a string value to document.cookie object:
document.cookie = "key1 = value1; key2 = value2; expires = date";
Q-BE28. How to read a cookie using JavaScript?
Reading a cookie is just as simple as writing one, because the value of the document.cookie object is the cookie. So you can use this string whenever you want to access the cookie.
The document.cookie string will keep a list of name = value pairs separated by semicolons, where name is the name of a cookie and value is its string value.
You can use strings’ split() function to break the string into key and values.
Q-BE29. How to delete a cookie using JavaScript?
If you want to delete a cookie so that subsequent attempts to read the cookie in JavaScript return nothing, you just need to set the expiration date to a time in the past. You should define the cookie path to ensure that you delete the right cookie. Some browsers will not let you delete a cookie if you don’t specify the path.
Q-BE30. Explain call(), apply() and, bind() methods
In JavaScript, the call(), apply(), and bind() methods are used to manipulate the execution context and binding of functions. They provide ways to explicitly set the value of this within a function and pass arguments to the function.
call(): The call() method allows you to invoke a function with a specified this value and individual arguments passed in as separate parameters. It takes the context object as the first argument, followed by the function arguments.
function greet(name) {
console.log(`Hello, ${name}! My name is ${this.name}.`);
}
const person = {
name: ‘John’
};
greet.call(person, ‘Alice’);
// Output: Hello, Alice! My name is John.
apply(): The apply() method is similar to call(), but it accepts arguments as an array or an array-like object. It also allows you to set the this value explicitly.
function greet(name, age) {
console.log(`Hello, ${name}! I am ${age} years old.`);
console.log(`My name is ${this.name}.`);
}
const person = {
name: ‘John’
};
greet.apply(person, [‘Alice’, 25]);
// Output: Hello, Alice! I am 25 years old.
// My name is John.
bind(): The bind() method creates a new function that has a specified this value and, optionally, pre-defined arguments. It allows you to create a function with a fixed execution context that can be called later.
function greet() {
console.log(`Hello, ${this.name}!`);
}
const person = {
name: ‘John’
};
const greetPerson = greet.bind(person);
greetPerson();
// Output: Hello, John!
In this example, bind() is used to create a new function greetPerson with the person object as the fixed execution context. When greetPerson() is called, it prints "Hello, John!".
The key difference between call(), apply(), and bind() lies in how they handle function invocation and argument passing. While call() and apply() immediately invoke the function, bind() creates a new function with the desired execution context but doesn’t invoke it right away.
These methods provide flexibility in manipulating function execution and context, enabling the creation of reusable functions and control over this binding in JavaScript.
Q-BE31. Explain call(), apply() and, bind() methods
call(): It’s a predefined method. This method invokes a method (function) by specifying the owner object:
function sayHello(){
return "Hello " + this.name;
}
var obj = {name: "Bruce"};
sayHello.call(obj);
// Returns "Hello Bruce"
Allows an object to use the method (function) of another object:
var person = {
age: 23,
getAge: function(){
return this.age;
}
}
var person2 = {age: 54};
person.getAge.call(person2);
// Returns 54
Accepts arguments:
function saySomething(message){
return this.name + " is " + message;
}
var person4 = {name: "John"};
saySomething.call(person4, "awesome");
// Returns "John is awesome"
apply(): The apply method is similar to the call() method. The only difference is that, call() method takes arguments separately whereas, apply() method takes arguments as an array.
function saySomething(message1, message2){
return this.name + " is " + message1 + " and " + message2;
}
var person4 = {name: "John"};
saySomething.apply(person4, ["awesome", "handsome"]);
// Returns "John is awesome and handsome"
bind(): This method returns a new function, where the value of "this" keyword will be bound to the owner object, which is provided as a parameter.
var bikeDetails = {
displayDetails: function(registrationNumber,brandName){
return this.name+ " , "+ "bike details: "+ registrationNumber + " , " + brandName;
}
}
var person1 = {name: "Alex"};
var detailsOfPerson1 = bikeDetails.displayDetails.bind(person1, "TS0122", "Grbike");
// Binds the displayDetails function to the person1 object
detailsOfPerson1();
//Returns Alex, bike details: TS0122, Grbike
Q-BE32. What is Hoisting in JavaScript?
Hoisting is a behavior in JavaScript where variable and function declarations are moved to the top of their respective scopes during the compilation phase, before the actual code execution takes place. This means that you can use variables and functions before they are declared in your code.
However, it is important to note that only the declarations are hoisted, not the initializations or assignments. So, while the declarations are moved to the top, any assignments or initializations remain in their original place.
In the case of variable hoisting, when a variable is declared using the var keyword, its declaration is hoisted to the top of its scope. This means you can use the variable before it is explicitly declared in the code. However, if you try to access the value of the variable before it is assigned, it will return undefined.
console.log(myVariable); // Output: undefined
let myVariable = 10;
console.log(myVariable); // Output: 10
In the above example, even though myVariable is accessed before its declaration, it doesn’t throw an error. However, the initial value is undefined until it is assigned the value of 10.
Function declarations are also hoisted in JavaScript. This means you can call a function before it is defined in the code. For example:
myFunction(); // Output: “Hello, World!”
function myFunction() {
console.log(“Hello, World!”);
}
In this case, the function declaration is hoisted to the top, so we can call myFunction() before its actual declaration.
It’s important to understand hoisting in JavaScript to avoid unexpected behavior and to write clean and maintainable code. It is recommended to declare variables and functions at the beginning of their respective scopes to make your code more readable and prevent potential issues related to hoisting.
Q-BE33. What is the differences between exec() and test() methods?
The exec() and test() methods are both used in JavaScript for working with regular expressions, but they serve different purposes.
exec() method: The exec() method is a regular expression method that searches a specified string for a match and returns an array containing information about the match or empty if no match is found. It returns an array where the first element is the matched substring, and subsequent elements provide additional information such as captured groups, index, and input string.
const regex = /hello (w+)/;
const str = ‘hello world’;
const result = regex.exec(str);
console.log(result);
// Output: [“hello world”, “world”, index: 0, input: “hello world”, groups: undefined]
In this example, the exec() method is used to match the regular expression /hello (w+)/ against the string "hello world". The resulting array contains the matched substring "hello world", the captured group "world", the index of the match, and the input string.
test() method: The test() method is also a regular expression method that checks if a pattern matches a specified string and returns a boolean value (true or false) accordingly. It simply indicates whether a match exists or not.
const regex = /hello/;
const str = ‘hello world’;
const result = regex.test(str);
console.log(result);
// Output: true
In this example, the test() method is used to check if the regular expression /hello/ matches the string "hello world". Since the pattern is found in the string, the method returns true.
In summary, the main difference between exec() and test() is that exec() returns an array with detailed match information or null, while test() returns a boolean value indicating whether a match exists or not. The exec() method is more suitable when you need to extract specific match details, while test() is useful for simple pattern verification.
Q-BE34. What is the difference between the var and let keywords in JavaScript?
The var and let keywords are both used to declare variables in JavaScript, but they have some key differences.
Scope:
The main difference between var and let is the scope of the variables they create. Variables declared with var have function scope, which means they are accessible throughout the function in which they are declared. Variables declared with let have block scope, which means they are only accessible within the block where they are declared.
For example, the following code will print the value of x twice, even though the second x is declared inside a block:
var x = 10;
{
var x = 20;
console.log(x); // 20
}
console.log(x); // 10
If we change the var keyword to let, the second x will not be accessible outside the block:
{
let x = 20;
console.log(x); // 20
}
console.log(x); // ReferenceError: x is not defined
Hoisting:
Another difference between var and let is that var declarations are hoisted, while let declarations are not. Hoisting means that the declaration of a variable is moved to the top of the scope in which it is declared, even though it is not actually executed until later.
For example, the following code will print the value of x even though the x declaration is not actually executed until the console.log() statement:
var x;
console.log(x); // undefined
x = 10;
If we change the var keyword to let, the console.log() statement will throw an error because x is not defined yet:
let x;
console.log(x); // ReferenceError: x is not defined
x = 10;
Redeclaration:
Finally, var declarations can be redeclared, while let declarations cannot. This means that you can declare a variable with the same name twice in the same scope with var, but you will get an error if you try to do the same with let.
For example, the following code will not cause an error:
var x = 10;
var x = 20;
console.log(x); // 20
However, the following code will cause an error:
let x = 10;
let x = 20;
// SyntaxError: x has already been declared
The var and let keywords are both used to declare variables in JavaScript, but they have some key differences in terms of scope, hoisting, and redeclaration. In general, it is recommended to use let instead of var, as it provides more predictable and reliable behavior.
Q-BE35. What is debugger in JavaScript?
Debugger in JavaScript is a tool or feature that helps writers find and fix mistakes in their code. It lets them stop the code from running, look at the variables, and analyze phrases to find bugs or strange behavior and fix them. The word debugger comes from the idea of getting rid of bugs or mistakes, which is how early engineers fixed problems with hardware.
Q-BE36. Explain Implicit Type Coercion in JavaScript
In JavaScript, implicit type coercion is when values are automatically changed from one data type to another while the code is running. It happens when numbers of different types are used in actions or comparisons. Implicit type coercion can be helpful, but it can also make code act in ways you didn’t expect, so it’s important to know how it works.
String coercion:
var x = 3;
var y = "3";
x + y // Returns "33"
Boolean coercion:
var x = 0;
var y = 23;
if(x) { console.log(x) } // The code inside this block will not run since the value of x is 0(Falsy)
if(y) { console.log(y) } // The code inside this block will run since the value of y is 23 (Truthy)
Logical operators:
var x = 220;
var y = "Hello";
var z = undefined;
x | | y // Returns 220 since the first value is truthy
x | | z // Returns 220 since the first value is truthy
x && y // Returns "Hello" since both the values are truthy
y && z // Returns undefined since the second value is falsy
Equality coercion:
var a = 12;
var b = "12";
a == b // Returns true because both 'a' and 'b' are converted to the same type and then compared. Hence the operands are equal.
var a = 226;
var b = "226";
a === b // Returns false because coercion does not take place and the operands are of different types. Hence they are not equal.
Here are the key rules of type coercion:
When a numeric value is concatenated with a string using the + operator, JavaScript coerces the number to a string.
When a string value is involved in an arithmetic operation, JavaScript attempts to convert it to a numeric value. ()
When comparing values using the == (loose equality) or != (loose inequality) operators, JavaScript performs type coercion to make the values comparable.
JavaScript has a concept of truthy and falsy values, where certain values are coerced to true or false in a boolean context.
Falsy values include false, 0, "" (empty string), null, undefined, and NaN. All other values are considered truthy when coerced to a boolean.
More info: https://medium.com/@atuljha2402/understanding-javascript-type-coercion-type-conversion-a2ce84c00331
Q-BE37. What is the difference between ViewState and SessionState?
ViewState is specific to a page in a session.
SessionState is specific to user-specific data that can be accessed across all web application pages.
Q-BE38. What does the following statement declare?
var myArray = [[[]]];
It declares a three-dimensional array.
Intermediate Questions
Q-IN1. What is the difference between Attributes and Property?
Attributes - provide more details on element like id, type, value etc.
Property - is the value assigned to the attribute like type = "text", value = "Name" etc.
Q-IN2. List out the different ways an HTML element can be accessed in a JavaScript code
Following are the list of ways:
getElementById(idname): Gets an element by its ID name.
getElementsByClass(classname): Gets all the elements that have the given classname.
getElementsByTagName(tagname): Gets all the elements that have the given tag name.
querySelector(): This function takes CSS style selector and returns the first selected element.
Q-IN3. How a JavaScript code can be involved in an HTML file?
There are 3 different ways in which a JavaScript code can be involved in an HTML file:
Inline
Internal
External
An inline function is a JavaScript function, which is assigned to a variable created at runtime. You can differentiate between Inline Functions and Anonymous since an inline function is assigned to a variable and can be easily reused. When you need a JavaScript for a function, you can either have the script integrated in the page you are working on, or you can have it placed in a separate file that you call, when needed. This is the difference between an internal script and an external script.
External script written in a separate js file, and then we link that file inside the <head> or <body> element of the HTML file where the code is to be placed.
Q-IN4. Explain High Order Functions in JavaScript
High-order functions in JavaScript are functions that can take other functions as inputs or return functions as their outputs. They make it possible to use strong functional programing methods that make code more flexible, reused, and expensive. By treating functions as first-class citizens, they make it possible to abstract behavior and make flexible code structures.
Q-IN5. What are object prototypes?
A prototype is a blueprint of an object. The prototype allows us to use properties and methods on an object even if the properties and methods do not exist on the current object.
var arr = [];
arr.push(2);
console.log(arr); // Outputs [2]
In the code above, as one can see, we have not defined any property or method called push on the array "arr" but the JS engine does not throw an error.
The reason is the use of prototypes. As we discussed before, Array objects inherit properties from the Array prototype.
The JS engine sees that the method push does not exist on the current array object and therefore, looks for the method push inside the Array prototype and it finds the method.
Q-IN6. How to define a variable in JavaScript?
There are 3 possible ways:
Var - The JavaScript variables statement is used to declare a variable and, optionally, we can initialize the value of that variable. Example: var a = 10; Variable declarations are processed before the execution of the code.
Const - The idea of const functions is not allow them to modify the object on which they are called. When a function is declared as const, it can be called on any type of object.
Let - It is a signal that the variable may be reassigned, such as a counter in a loop, or a value swap in an algorithm. It also signals that the variable will be used only in the block it’s defined in.
Q-IN7. What is the role of break and continue statements?
The break statement is used to come out of the current loop. In contrast, the continue statement continues the current loop with a new recurrence.
Q-IN8. What is Typed language?
Typed Language is in which the values are associated with values and not with variables. It is of two types:
Dynamically: in this, the variable can hold multiple types; like in JS a variable can take number, chars. JavaScript is a loosely (dynamically) typed language, variables in JS are not associated with any type
Statically: in this, the variable can hold only one type, like in Java a variable declared of string can take only set of characters and nothing else.
Q-IN9. What is the difference between Local Storage & Session Storage?
Local Storage - The data is not sent back to the server for every HTTP request (HTML, images, JavaScript, CSS, etc) – reducing the amount of traffic between client and server. It will stay until it is manually cleared through settings or program.
Session Storage - It is similar to local storage; the only difference is while data stored in local storage has no expiration time, data stored in session storage gets cleared when the page session ends. Session Storage will leave when the browser is closed.
Q-IN10. What is the difference between the operators == & ===?
The main difference between "==" and "===" operator is that formerly compares variable by making type correction e.g. if you compare a number with a string with numeric literal, == allows that, but === doesn’t allow that, because it not only checks the value but also type of two variable, if two variables are not of the same type "===" return false, while "==" return true.
Q-IN11. What is currying in JavaScript?
Currying is a JavaScript functional programming approach that converts a function with many parameters into a succession of functions that each take one argument. It allows you to use only a portion of the inputs, allowing you to create functions that may be used several times and are specialized.
It transforms a function of arguments n, to n functions of one or fewer arguments.
function multiply(a,b){
return a*b;
}
function currying(fn){
return function(a){
return function(b){
return fn(a,b);
}
}
}
var curriedMultiply = currying(multiply);
multiply(4, 3); // Returns 12
curriedMultiply(4)(3); // Also returns 12
As one can see in the code above, we have transformed the function multiply(a,b) to a function curriedMultiply, which takes in one parameter at a time.
Q-IN12. What is the difference between null and undefined?
Conceptually, undefined indicates the absence of a value, while null indicates the absence of an object.
Q-IN13. What is the difference between undeclared and undefined?
Undeclared variables are those that do not exist in a program and are not declared. If the program tries to read the value of an undeclared variable, then a runtime error is encountered. Undefined variables are those that are declared in the program but have not been given any value. If the program tries to read the value of an undefined variable, an undefined value is returned.
Q-IN14. Name some JavaScript frameworks
A JavaScript framework is an application framework written in JavaScript. It differs from a JavaScript library in its control flow. There are many JavaScript Frameworks available but some of the most commonly used frameworks are:
Q-IN15. What is the difference between window and document in JavaScript?
Window: JavaScript window is a global object which holds variables, functions, history, location.
Document: The document also comes under the window and can be considered as the property of the window.
Q-IN16. What is the use of void (0)?
void(0) is used to prevent the page from refreshing, and parameter “zero” is passed while calling.
void(0) is used to call another method without refreshing the page.
Q-IN17. What are all the types of Pop up boxes available in JavaScript?
Alert
Confirm and
Prompt
Q-IN18. What is the difference between an alert box and a confirmation box?
Alert box displays only one button, which is the OK button.
Confirmation box displays two buttons, namely OK and cancel.
Q-IN19. What is the difference between innerHTML and innerText?
innerHTML: it will process an HTML tag if found in a string.
innerText: it will not process an HTML tag if found in a string.
Q-IN20. What are the disadvantages of using innerHTML in JavaScript?
If you use innerHTML in JavaScript, the disadvantage is:
Content is replaced everywhere.
We cannot use it like appending to innerHTML.
Even if you use += like "innerHTML = innerHTML + 'html'" still the old content is replaced by html.
The entire innerHTML content is re-parsed and builds into elements. And innerHTML content is refreshed every time and thus is slower. Therefore, it’s much slower.
The innerHTML does not provide validation, and therefore we can potentially insert valid and broken HTML in the document and break it.
Q-IN21. Which keywords are used to handle exceptions?
try - catch - finally are used to handle exceptions in the JavaScript:
try {
// Try some code
}
catch(exception) {
// Catch exception and code what to do next
}
finally {
// Code runs either it finishes successfully or after catch
}
Q-IN22. What is unescape() and escape() functions?
The escape() function is responsible for coding a string to transfer the information from one computer to the other across a network.
<script>
document.write(escape("Hello? How are you!"));
</script>
// Output: Hello%3F%20How%20are%20you%21
The unescape() function is very important as it decodes the coded string.
It works in the following way.
<script>
document.write(unescape("Hello%3F%20How%20are%20you%21"));
</script>
// Output: Hello? How are you!
Q-IN23. What are the decodeURI() and encodeURI()?
encodeURl() is used to convert URL into their hex coding. And decodeURI() is used to convert the encoded URL back to normal.
<script>
var uri="my test.asp?name=ståle&car=saab";
document.write(encodeURI(uri)+ "<br>");
document.write(decodeURI(uri));
</script>
// Output
my%20test.asp?name=st%C3%A5le&car=saab
my test.asp?name=ståle&car=saab
Q-IN24. What is an event bubbling in JavaScript?
Event bubbling is a way of event propagation in the HTML DOM API, when an event occurs in an element inside another element, and both elements have registered a handle for that event. With bubbling, the event is first captured and handled by the innermost element and then propagated to outer elements. The execution starts from that event and goes to its parent element. Then the execution passes to its parent element and so on till the body element.
In short, JavaScript allows DOM elements to be nested inside each other. In such a case, if the handler of the child is clicked, the handler of the parent will also work as if it were clicked too.
Q-IN25. What is NaN in JavaScript?
NaN is a short form of Not a Number. Since NaN always compares unequal to any number, including NaN, it is usually used to indicate an error condition for a function that should return a valid number. When a string or something else is being converted into a number and that cannot be done, then we get to see NaN.
Q-IN26. How do JavaScript primitive/object types passed in functions?
One of the differences between the two is that Primitive Data Types are passed By Value and Objects are passed By Reference.
By Value means creating a COPY of the original. Picture it like twins: they are born exactly the same, but the first twin can have short hair whereas the other has long hair.
By Reference means creating an ALIAS to the original. When your Mom calls you “Pumpkin Pie” although your name is Margaret, this doesn’t suddenly give birth to a clone of yourself: you are still one, but these two very different names can call you.
Q-IN27. How can you convert the string of any base to integer in JavaScript?
The parseInt() function is used to convert numbers between different bases. It takes the string to be converted as its first parameter, and the second parameter is the base of the given string.
parseInt("4F", 16)
Q-IN28. What would be the result of 2+5+"3"?
Since 2 and 5 are integers, they will be added numerically. And since 3 is a string, its concatenation will be done. So the result would be 73. The "" makes all the difference here and represents 3 as a string and not a number.
Q-IN29. What are Exports & Imports?
Imports and exports help us to write modular JavaScript code. Using Imports and exports we can split our code into multiple files.
//------ lib.js ------
export const sqrt = Math.sqrt;
export function square(x) {
return x * x;
}
export function diag(x, y) {
return sqrt(square(x) + square(y));
}
//------ main.js ------
import { square, diag } from 'lib';
console.log(square(5)); // 25
console.log(diag(4, 3)); // 5
Q-IN30. What are the different types of errors in JavaScript?
There are three types of errors:
Load time errors: Errors that come up when loading a web page, like improper syntax errors, are known as Load time errors and generate the errors dynamically.
Runtime errors: Errors that come due to misuse of the command inside the HTML language. This is the error that comes up while the program is running. For example, illegal operations cause the division of a number by zero or access a non-existent area of the memory.
Logical errors: These are the errors that occur due to the bad logic performed on a function with a different operation. It is caused by syntactically incorrect code, which does not fulfill the required task - for example, an infinite loop.
Q-IN31. What is memoization?
Memoization is a form of caching where the return value of a function is cached based on its parameters. If the parameter of that function is not changed, the cached version of the function is returned.
Let’s understand memoization, by converting a simple function to a memoized function:
function addTo256(num){
return num + 256;
}
addTo256(20); // Returns 276
addTo256(40); // Returns 296
addTo256(20); // Returns 276
Computing the result with the same parameter, again and again, is not a big deal in the above case, but imagine if the function does some heavy-duty work, then, computing the result again and again with the same parameter will lead to wastage of time.
This is where memoization comes in, by using memoization we can store(cache) the computed results based on the parameters. If the same parameter is used again while invoking the function, instead of computing the result, we directly return the stored (cached) value.
Let’s convert the above function addTo256, to a memoized function:
function memoizedAddTo256(){
var cache = {};
return function(num){
if(num in cache){
console.log("cached value");
return cache[num]
} else{
cache[num] = num + 256;
return cache[num];
}
}
}
var memoizedFunc = memoizedAddTo256();
memoizedFunc(20); // Normal return
memoizedFunc(20); // Cached return
In the code above, if we run the memoizedFunc function with the same parameter, instead of computing the result again, it returns the cached result. Although using memoization saves time, it results in larger consumption of memory since we are storing all the computed results.
Q-IN32. What is recursion in a programing language?
Recursion is a technique to iterate over an operation by having a function call itself repeatedly until it arrives at a result.
function add(number) {
if (number <= 0) {
return 0;
} else {
return number + add(number - 1);
}
}
add(3) => 3 + add(2)
3 + 2 + add(1)
3 + 2 + 1 + add(0)
3 + 2 + 1 + 0 = 6
Q-IN33. What is the use of a constructor function in JavaScript?
Constructor functions are used to create objects in JS. If we want to create multiple objects having similar properties and methods, constructor functions are used.
The name of a constructor function should always be written in Pascal Notation: every word should start with a capital letter.
function Person(name,age,gender){
this.name = name;
this.age = age;
this.gender = gender;
}
var person1 = new Person("Vivek", 76, "male");
console.log(person1);
var person2 = new Person("Courtney", 34, "female");
console.log(person2);
In the code above, we have created a constructor function named Person. Whenever we want to create a new object of the type Person, we need to create it using the new keyword.
Q-IN34. What is DOM and how are DOM utilized in JavaScript?
DOM stands for Document Object Model. DOM is a programming interface for HTML and XML documents.
When the browser tries to render an HTML document, it creates an object based on the HTML document called DOM. Using this DOM, we can manipulate or change various elements inside the HTML document.
It is responsible for how various objects in a document interact with each other. DOM is required for developing web pages, which includes objects like paragraphs, links, etc. The web browser creates a DOM of the webpage when the page is loaded. These objects can be operated to include actions like add or delete. DOM is also required to add extra capabilities to a web page.
Q-IN35. What is BOM?
Browser Object Model is known as BOM. It allows users to interact with the browser. A browser's initial object is a window. As a result, you may call all of the window's functions directly or by referencing the window. The document, history, screen, navigator, location, and other attributes are available in the window object.
Q-IN36. How are event handlers utilized in JavaScript?
Events are the actions that result from activities, such as clicking a link or filling a form by the user. An event handler is required to manage the proper execution of all these events. Event handlers are an extra attribute of the object. This attribute includes the event’s name and the action taken if the event takes place.
Q-IN37. What is the role of deferred scripts in JavaScript?
The HTML code’s parsing during page loading is paused by nature until the script hasn't halted. If the server is slow or the script is particularly heavy, then the web page is delayed.
While using Deferred, scripts delays execution of the script till the time the HTML parser finished. This reduces the loading time of web pages, and they get displayed faster.
Q-IN38. How are JavaScript and ECMA Script related?
ECMA Script is like rules and guidelines, while JavaScript is a scripting language used for web development.
Advanced Questions
Q-AD1. What are arrow functions?
Arrow functions were introduced in ES6. They provide us with a new and shorter syntax for declaring functions. Arrow functions can only be used as a function expression.
// Traditional Function Expression
var add = function(a,b){
return a + b;
}
// Arrow Function Expression
var arrowAdd = (a,b) => a + b;
Arrow functions are:
Declared without the function keyword.
If there is only one returning expression then we don’t need to use the return keyword.
For functions having just one line of code, curly braces { } can be omitted.
If the function takes in only one argument, then the parenthesis () around the parameter can be omitted as shown in the code above.
The biggest difference between the traditional function expression and the arrow function is the handling of this keyword:
var obj1 = {
valueOfThis: function(){
return this;
}
}
var obj2 = {
valueOfThis: () => {
return this;
}
}
obj1.valueOfThis(); // Will return the object obj1
obj2.valueOfThis(); // Will return window/global object
By general definition, this keyword always refers to the object that is calling the function. As you can see in the code above, obj1.valueOfThis() returns obj1 since this keyword refers to the object calling the function.
In the arrow functions, there is no binding of this keyword. This keyword inside an arrow function does not refer to the object calling it. It rather inherits its value from the parent scope which is the window object in this case. Therefore, in the code above, obj2.valueOfThis() returns the window object.
Q-AD2. What do mean by prototype design pattern?
The Prototype Pattern produces different objects, but instead of returning uninitialized objects, it produces objects that have values replicated from a template – or sample – object. Also known as the Properties pattern, the Prototype pattern is used to create prototypes.
The introduction of business objects with parameters that match the database's default settings is a good example of where the Prototype pattern comes in handy. The default settings for a newly generated business object are stored in the prototype object.
The Prototype pattern is hardly used in traditional languages, however, it is used in the development of new objects and templates in JavaScript, which is a prototypal language.
Q-AD3. Differences between declaring variables using var, let and const
Before the ES6 version of JS, only the keyword var was used to declare variables. With the ES6 Version, keywords let and const were introduced to declare variables.
keyword | const | let | var |
global scope | no | no | yes |
function scope | yes | yes | yes |
block scope | yes | yes | no |
can be reassigned | no | yes | yes |
Example of var vs let in global scope:
var variable1 = 23;
let variable2 = 89;
function catchValues(){
console.log(variable1);
console.log(variable2);
// Both the variables can be accessed anywhere since they are declared in the global scope
}
window.variable1; // Returns the value 23
window.variable2; // Returns undefined
The let keyword in the global scope behave just like the var keyword in the global scope.
Both can be accessed anywhere in the code.
One difference: Variables that are declared with the var keyword in the global scope are added to the window/global object. Therefore, they can be accessed using window.variableName. Whereas, the variables declared with the let keyword are not added to the global object, therefore, trying to access such variables using window.variableName results in an error.
Example of var vs let in function scope:
function varVsLetFunction(){
let awesomeCar1 = "Audi";
var awesomeCar2 = "Mercedes";
}
console.log(awesomeCar1); // Throws an error
console.log(awesomeCar2); // Throws an error
Variables are declared in a functional/local scope using var and let keywords behave exactly the same, meaning, they cannot be accessed from outside of the scope.
Example of var vs let in block scope:
{
var variable3 = [1, 2, 3, 4];
}
console.log(variable3); // Outputs [1,2,3,4]
{
let variable4 = [6, 55, -1, 2];
}
console.log(variable4); // Throws error
for(let i = 0; i < 2; i++){
//Do something
}
console.log(i); // Throws error
for(var j = 0; j < 2; i++){
// Do something
}
console.log(j) // Outputs 2
In JS, a block means the code written inside the curly braces {}.
Variables declared with var keyword do not have block scope. It means a variable declared in block scope {} with the var keyword is the same as declaring the variable in the global scope.
Variables declared with let keyword inside the block scope cannot be accessed from outside of the block.
Const:
Variables with the const keyword behave exactly like a variable declared with the let keyword with only one difference, any variable declared with the const keyword cannot be reassigned.
const x = {name:"Vivek"};
x = {address: "India"}; // Throws an error
x.name = "Nikhil"; // No error is thrown
const y = 23;
y = 44; // Throws an error
In the code above, although we can change the value of a property inside the variable declared with const keyword, we cannot completely reassign the variable itself.
Q-AD4. What is the rest parameter and spread operator?
Both rest parameter and spread operator were introduced in the ES6.
Rest parameter ( … ):
Provides an improved way of handling the parameters of a function.
We can create functions that can take a variable number of arguments.
Number of arguments will be converted into an array using the rest parameter.
It also helps in extracting all or some parts of the arguments.
function extractingArgs(...args){
return args[1];
}
extractingArgs(8,9,1); // Returns 9
function addAllArgs(...args){
let sumOfArgs = 0;
let i = 0;
while(i < args.length){
sumOfArgs += args[i];
i++;
}
return sumOfArgs;
}
addAllArgs(6, 5, 7, 99); // Returns 117
addAllArgs(1, 3, 4); // Returns 8
Rest parameter should always be used at the last parameter of a function:
// Incorrect way to use rest parameter
function randomFunc(a,...args,c){
//Do something
}
// Correct way to use rest parameter
function randomFunc2(a,b,...args){
//Do something
}
Spread operator (…):
Although the syntax of the spread operator is exactly the same as the rest parameter, the spread operator is used to spreading an array, and object literals. We also use spread operators where one or more arguments are expected in a function call.
function addFourNumbers(num1,num2,num3,num4){
return num1 + num2 + num3 + num4;
}
let fourNumbers = [5, 6, 7, 8];
addFourNumbers(...fourNumbers);
// Spreads [5,6,7,8] as 5,6,7,8
let array1 = [3, 4, 5, 6];
let clonedArray1 = [...array1];
// Spreads the array into 3,4,5,6
console.log(clonedArray1); // Outputs [3,4,5,6]
let obj1 = {x:'Hello', y:'Bye'};
let obj2 = {z:'Yes', a:'No'};
let mergedObj = {...obj1, ...obj2}; // Spreads both the objects and merges it
console.log(mergedObj);
// Outputs {x:'Hello', y:'Bye', z:'Yes', a:'No'};
Key differences between rest parameter and spread operator:
Rest parameter is used to take a variable number of arguments and turns them into an array while the spread operator takes an array or an object and spreads it
Rest parameter is used in function declaration whereas the spread operator is used in function calls.
Q-AD5. How many different methods can we create an object?
Object Literals:
Object literals are a comma-separated list of key-value pairs wrapped in curly braces
const user = {
firstName: "John",
lastName: "Baker",
getFullName: function() {
return this.firstName + this.lastName;
}
};
Constructor Functions:
We define an object type without any specific values. Then, we create new object instances and populate each of them with different values.
function User(firstName, lastName) {
this.firstName = firstName,
this.lastName = lastName,
this.getFullName = function() {
return this.firstName + this.lastName;
}
};
const user = new User("John", "Baker");
ECMAScript 6 Classes:
ECMAScript 6 introduced the class keyword to create classes in JavaScript. Now we can use the class attribute to create a class in JavaScript instead of a function constructor, and use the new operator to create an instance.
class User {
constructor(firstName, lastName) {
this.firstName = firstName,
this.lastName = lastName,
this.getFullName = function() {
return this.firstName + this.lastName;
}
}
};
const user = new User("John", "Baker");
Object.create Method:
We can also create new objects using the Object.create() method, which allows us to use an existing object literal as the prototype of a new object we create.
const user = {
firstName: "John",
lastName: "Baker",
getFullName: function() {
return this.firstName + this.lastName;
}
};
const anotherUser = Object.create(user);
console.log(anotherUser.firstName); // Output: John
anotherUser.firstName = "Jack";
console.log(anotherUser.firstName); // Output: Jack
Q-AD6. What is the ‘Strict’ mode in JavaScript and how can it be enabled?
Strict mode is a way to introduce better error-checking into your code.
When you use strict mode, you cannot use implicitly declared variables, or assign a value to a read-only property, or add a property to an object that is not extensible.
You won't be able to use the JavaScript keyword as a parameter or function name.
You will not be allowed to create global variables
You can enable strict mode by adding "use strict" at the beginning of a file, a program, or a function.
Q-AD7. What is a prompt box in JavaScript?
A prompt box is a box that allows the user to enter input by providing a text box. The prompt() method displays a dialog box that prompts the visitor for input. A prompt box is typically used when you want the user to put a value before looking at a page. To move further, the user must click "OK" or "Cancel" in the prompt box that displays after entering an input value.
Q-AD8. What is a window.onload and onDocumentReady?
The onload function is not run until all the information on the page is loaded. This leads to a substantial delay before any code is executed.
onDocumentReady loads the code just after the DOM is loaded. This allows early manipulation of the code.
Q-AD9. Explain passed by value and passed by reference
In JavaScript, primitive data types when passed to another variable, are passed by value. Instead of just assigning the same address to another variable, the value is passed and new space of memory is created.
var y = 2; // y pointing to address #1347
var z = y; // z pointing to a completely new address of the value 2: #2589
// Changing the value of y
y = 3;
console.log(z); // Returns 2, since z points to a new address in the memory so changes in y will not effect z
While passing non-primitive data types, the assigned operator directly passes the address (reference).
var obj = {name: "Batman"}; // obj pointing to address of object #1347
var obj2 = obj; // obj2 pointing to the same address
// changing the value of obj1
obj.name = "Superman";
console.log(obj2);
// Returns {name:"Superman"} since both the variables are pointing to the same address.
Q-AD10. What is namespacing in JavaScript, and how is it used?
Namespacing is used for grouping the desired functions, variables, etc., under a unique name. It is a name that has been attached to the desired functions, objects, and properties.
// Creating a namespace called 'MyApp'
let MyApp = MyApp || {};
// Adding functionality to the namespace
MyApp.calculator = {
add: function (a, b) {
return a + b;
},
subtract: function (a, b) {
return a - b;
}
};
MyApp.logger = {
log: function (message) {
console.log(message);
}
};
// Using the functionality from the namespace
var result = MyApp.calculator.add(5, 3);
MyApp.logger.log('Result: ' + result);
// Output: 8
Preventing Global Scope Pollution: Prevents variables and functions from polluting the global scope by encapsulating them within a namespace.
Organization and Modularity: It allows you to group related functions and variables under a common namespace, making the codebase more structured and easier to manage.
Encapsulation: Restricts access to variables and functions within the namespace. Helps in avoiding unintended external interference and promotes information hiding.
Readability and Maintenance: Developers can easily understand the context of code inside a namespace, making it easier to navigate and update code.
Reducing Naming Conflicts: Helps mitigate these conflicts by isolating code within its designated namespace.
Q-AD11. What is closure?
Closures are an ability of a function to remember the variables and functions that are declared in its outer scope.
Closures are created whenever a variable that is defined outside the current scope is accessed from within some inner scope.
var Person = function(pName){
var name = pName
this.getName = function(){
return name;
}
}
var person = new Person("Neelesh");
console.log(person.getName()); // Output: Neelesh
This ability of a function to store a variable for further reference even after it is executed is called Closure.
More info: https://javascript.info/closure
Q-AD12. How closures work in JavaScript?
The closure is a locally declared variable related to a function that stays in memory when it has returned.
Example:
function greeting() {
let message = 'Hi';
function sayHi() {
console.log(message);
}
return sayHi;
}
let hi = greeting();
hi(); // still can access the message variable. Output: Hi
However, the interesting point here is that, typically, a local variable only exists during the function’s execution.
It means that when the greeting() function has completed executing, the message variable is no longer accessible.
In this case, we execute the hi() function that references the sayHi() function, the message variable still exists.
The magic of this is closure. In other words, the sayHi() function is a closure.
Another example:
function greeter(name, age) {
var message = name + " says howdy!! He is " + age + " years old";
return function greet() {
console.log(message);
};
}
// Generate the closure
var JamesGreeter = greeter("James", 23);
// Use the closure
JamesGreeter(); // Output: James says howdy!! He is 23 years old
Q-AD13. What is the use of promises?
Promises are used to handle asynchronous operations in JS. Before promises, callbacks were used to handle asynchronous operations. But due to the limited functionality of callbacks, using multiple callbacks to handle asynchronous code can lead to unmanageable code.
Promise object has four states:
Pending - Initial state: Represents that the promise has neither been fulfilled nor been rejected.
Fulfilled: Represents that the promise has been fulfilled, meaning the async operation is completed.
Rejected: Represents that the promise has been rejected for some reason, meaning the async operation has failed.
Settled: Represents that the promise has been either rejected or fulfilled.
A promise is created using the Promise constructor which takes in a callback function with two parameters, resolve and reject respectively:
resolve is a function that will be called when the async operation has been successfully completed.
reject is a function that will be called, when the async operation fails or if some error occurs.
Example:
function sumOfThreeElements(...elements){
return new Promise((resolve,reject)=>{
if(elements.length > 3 ){
reject("Only three elements or less are allowed");
}
else{
let sum = 0;
let i = 0;
while(i < elements.length){
sum += elements[i];
i++;
}
resolve("Sum has been calculated: "+sum);
}
})
}
We can consume any promise by attaching then() and catch() methods to the consumer.
then() method is used to access the result when the promise is fulfilled.
catch() method is used to access the result/error when the promise is rejected.
sumOfThreeElements(4, 5, 6)
.then(result=> console.log(result))
.catch(error=> console.log(error));
// In the code above, the promise is fulfilled so the then() method gets executed
sumOfThreeElements(7, 0, 33, 41)
.then(result => console.log(result))
.catch(error=> console.log(error));
// In the code above, the promise is rejected hence the catch() method gets executed
Q-AD14. What are classes in JS?
Introduced in the ES6 version, classes are nothing but syntactic sugars for constructor functions.
// Before ES6 version, using constructor functions
function Student(name, rollNumber, grade, section){
this.name = name;
this.rollNumber = rollNumber;
this.grade = grade;
this.section = section;
}
// Way to add methods to a constructor function
Student.prototype.getDetails = function(){
return 'Name: ${this.name}, Roll no: ${this.rollNumber}, Grade: ${this.grade}, Section:${this.section}';
}
let student1 = new Student("Vivek", 354, "6th", "A");
student1.getDetails();
// Returns Name: Vivek, Roll no:354, Grade: 6th, Section:A
// ES6 version classes
class Student{
constructor(name,rollNumber,grade,section){
this.name = name;
this.rollNumber = rollNumber;
this.grade = grade;
this.section = section;
}
// Methods can be directly added inside the class
getDetails(){
return 'Name: ${this.name}, Roll no: ${this.rollNumber}, Grade:${this.grade}, Section:${this.section}';
}
}
let student2 = new Student("Garry", 673, "7th", "C");
student2.getDetails();
// Returns Name: Garry, Roll no: 673, Grade: 7th, Section: C
Keys points to remember:
Unlike functions, classes are not hoisted. A class cannot be used before it is declared.
A class can inherit properties and methods from other classes by using the extend keyword.
All the syntaxes inside the class must follow the strict mode(‘use strict’) of JS. An error will be thrown if the strict mode rules are not followed.
Q-AD15. What are generator functions?
Generator functions are a special class of functions. They can be stopped midway and then continue from where they had stopped.
function* genFunc(){
// Perform operation
}
In the case of generator functions, when called, they do not execute the code, instead, they return a generator object. This generator object handles the execution.
function* genFunc(){
yield 3;
yield 4;
}
genFunc(); // Returns Object [Generator] {}
The generator object consists of a method called next(), this method when called, executes the code until the nearest yield statement, and returns an object consisting of a value and done properties.
function* iteratorFunc() {
let count = 0;
for (let i = 0; i < 2; i++) {
count++;
yield i;
}
return count;
}
let iterator = iteratorFunc();
console.log(iterator.next()); // {value:0,done:false}
console.log(iterator.next()); // {value:1,done:false}
console.log(iterator.next()); // {value:2,done:true}
Q-AD16. Explain WeakSet in JS
In JS, a Set is a collection of unique and ordered elements. Just like Set, WeakSet is also a collection of unique and ordered elements with some key differences:
Weakset contains only objects and no other type.
An object inside the weakset is referenced weakly. This means, if the object inside the weakset does not have a reference, it will be garbage collected.
Unlike Set, WeakSet only has three methods, add() , delete() and has().
const newSet = new Set([4, 5, 6, 7]);
console.log(newSet);// Outputs Set {4,5,6,7}
const newSet2 = new WeakSet([3, 4, 5]); //Throws an error
let obj1 = {message:"Hello world"};
const newSet3 = new WeakSet([obj1]);
console.log(newSet3.has(obj1)); // true
Q-AD17. Explain WeakMap in JS
In JS, Map is used to store key-value pairs. The key-value pairs can be of both primitive and non-primitive types. WeakMap is similar to Map with key differences:
The keys and values in WeakMap should always be an object.
If there are no references to the object, the object will be garbage collected.
const map1 = new Map();
map1.set('Value', 1);
const map2 = new WeakMap();
map2.set('Value', 2.3); // Throws an error
let obj = {name:"Vivek"};
const map3 = new WeakMap();
map3.set(obj, {age:23});
Q-AD18. What is Object Destructuring?
Object destructuring is a new way to extract elements from an object or an array.
const classDetails = {
strength: 78,
benches: 39,
blackBoard: 1
}
const {strength:classStrength, benches:classBenches, blackBoard:classBlackBoard} = classDetails;
console.log(classStrength); // Outputs 78
console.log(classBenches); // Outputs 39
console.log(classBlackBoard); // Outputs 1
If we want our new variable to have the same name as the property of an object we can remove the colon:
const {strength:strength} = classDetails;
// The above line of code can be written as:
const {strength} = classDetails;
The same usage can be done for array destructuring:
const arr = [1, 2, 3, 4];
const [first, second, third, fourth] = arr;
console.log(first); // Outputs 1
console.log(second); // Outputs 2
console.log(third); // Outputs 3
console.log(fourth); // Outputs 4
Q-AD19. What is a Temporal Dead Zone?
Temporal Dead Zone is a behaviour that occurs with variables declared using let and const keywords. It is a behaviour where we try to access a variable before it is initialized. Examples of temporal dead zone:
x = 23; // Gives reference error
let x;
function anotherRandomFunc(){
message = "Hello"; // Throws a reference error
let message;
}
anotherRandomFunc();
In the code above, both in the global scope and functional scope, we are trying to access variables that have not been declared yet. This is called the Temporal Dead Zone.
Q-AD20. What do you mean by JavaScript Design Patterns?
JavaScript design patterns are repeatable approaches for errors that arise sometimes when building JavaScript browser applications. They truly assist us in making our code more stable.
They are divided mainly into 3 categories:
Creational Design Pattern: The object generation mechanism is addressed by the JavaScript Creational Design Pattern. They aim to make items that are appropriate for a certain scenario.
Structural Design Pattern: This explains how the classes and objects we've generated so far can be combined to construct bigger frameworks. This pattern makes it easier to create relationships between items by defining a straightforward way to do so.
Behavioral Design Pattern: This design pattern highlights typical patterns of communication between objects in JavaScript. As a result, the communication may be carried out with greater freedom.
Q-AD21. Is JavaScript a pass-by-reference or pass-by-value language?
The variable's data is always a reference for objects, hence JavaScript is always pass by value. As a result, if you supply an object and alter its members inside the method, the changes continue outside of it. It appears to be pass by reference in this case. However, if you modify the values of the object variable, the change will not last, demonstrating that it is indeed passed by value.
Q-AD22. What is the difference between Call and Apply?
The call() method calls a function with a given this value and arguments provided individually.
fun.call(thisArg[, arg1[, arg2[, ...]]])
The apply() method calls a function with a given this value, and arguments provided as an array.
fun.apply(thisArg, [argsArray])
Q-AD23. How to empty an Array in JavaScript?
There are a number of methods you can use to empty an array:
Method 1:
arrayList = []
Above code will set the variable arrayList to a new empty array. This is recommended if you don’t have references to the original array arrayList anywhere else, because it will actually create a new, empty array. You should be careful with this method of emptying the array, because if you have referenced this array from another variable, then the original reference array will remain unchanged.
Method 2:
arrayList.length = 0;
The code above will clear the existing array by setting its length to 0. This way of emptying the array also updates all the reference variables that point to the original array. Therefore, this method is useful when you want to update all reference variables pointing to arrayList.
Method 3:
arrayList.splice(0, arrayList.length);
The implementation above will also work perfectly. This way of emptying the array will also update all the references to the original array.
Method 4:
while(arrayList.length){
arrayList.pop();
}
The implementation above can also empty arrays, but it is usually not recommended to use this method often.
Q-AD24. What do you mean by Self Invoking Functions?
JavaScript functions that execute instantly upon definition without requiring an explicit function call are referred to as self-invoking functions, or immediately invoked function expressions (IIFE).
When you create a function that invokes itself, you may use parentheses or other operators to invoke it immediately after enclosing the function declaration or expression in parenthesis. A self-invoking function looks like this:
(function() {
// Function body
})();
In this example, the function is defined inside parentheses (function() { … }). The trailing parentheses `()` immediately invoke the function. Because it lacks a reference to be called again, the function is only ever run once before being deleted.
In order to keep variables, functions, or modules out of the global namespace, self-invoking functions are frequently used to establish a distinct scope. Code enclosed in a self-invoking function allows you to define variables without worrying about them clashing with other variables in the global scope.
Using a self-invoking procedure to establish a private variable is illustrated in the following example:
(function() {
var privateVariable = ‘This is private’;
// Other code within the function
})();
This means that the variable {privateVariable} cannot be viewed or changed from outside of the self-invoking function and can only be accessed within its scope.
Another technique to run code instantly and give you a chance to assign the result to a variable or return values is by using self-invoking functions:
var result = (function() {
// Code logic
return someValue;
})();
The outcome of the self-invoking function is designated to the result variable in this instance. This preserves a clear separation of scope and lets you carry out computations, initialize variables, or run code immediately.
Self-invoking functions offer a useful technique for creating isolated scopes and executing code immediately without cluttering the global scope. They are commonly used in modular patterns to avoid naming collisions or leaking variables.
Q-AD25. What has to be done in order to put Lexical Scoping into practice?
To support lexical scoping, a JavaScript function object's internal state must include not just the function's code but also a reference to the current scope chain.
Q-AD26. What is the reason for wrapping the entire content of a JavaScript source file in a function book?
This is an increasingly common practice, employed by many popular JavaScript libraries. This technique creates a closure around the entire contents of the file which, perhaps most importantly, creates a private namespace and thereby helps avoid potential name clashes between different JavaScript modules and libraries. Another feature of this technique is to allow for an easy alias for a global variable. This is often used in jQuery plugins.
Q-AD27. What are escape characters in JavaScript?
JavaScript escape characters enable you to write special characters without breaking your application. Escape characters (Backslash) is used when working with special characters like single quotes, double quotes, apostrophes and ampersands. Place backslash before the characters to make it display.
console.log("I am a \"good\" boy")
// Output: "I am a "good" boy"
Q-AD28. What is JavaScript Unit Testing, and what are the challenges in JavaScript Unit Testing?
JavaScript Unit Testing is a testing method in which JavaScript tests code written for a web page or web application module. It is combined with HTML as an inline event handler and executed in the browser to test if all functionalities work fine. These unit tests are then organized in the test suite.
Every suite contains several tests designed to be executed for a separate module.
Challenges:
Many other languages support unit testing in browsers, in the stable as well as in runtime environment, but JavaScript can not.
You can understand some system actions with other languages, but this is not the case with JavaScript.
Some JavaScript are written for a web application that may have multiple dependencies.
Difficulties with page rendering and DOM manipulation.
Solutions:
Do not use global variables.
Do not manipulate predefined objects.
Design core functionalities based on the library.
Try to create small pieces of functionalities with lesser dependencies.
Q-AD29. What are some important JavaScript Unit Testing Frameworks?
Unit.js: An open-source assertion library running on browser and Node.js. It is compatible with other JavaScript Unit Testing frameworks like Mocha, Karma, Jasmine, QUnit, Protractor, etc.
QUnit: Supports both client-side and server-side JavaScript Unit Testing for jQuery projects. It follows Common JS unit testing and supports the Node Long-term Support Schedule.
Jasmine: Behavior-driven development framework. It is used for testing both synchronous and asynchronous JavaScript codes. It does not require DOM and comes with an easy syntax that can be written for any test.
Karma: An open-source productive testing environment. Easy workflow control running on the command line. Test can be run on real devices with easy debugging.
Mocha: Runs on Node.js and in the browser, supports asynchronous testing with accuracy and flexibility in reporting. Provides tremendous support of rich features such as test-specific timeouts, JavaScript APIs.
Jest: Provides the ‘zero-configuration testing experience and supports independent and non-interrupting running tests without any conflict. it does not require any other setup configuration and libraries - used by Facebook.
AVA: Tests are being run in parallel and serially. It supports asynchronous testing as well. AVA uses sub-processes to run the unit test.
Coding Questions
Q-CO1. What will be the output of the following code?
//nfe (named function expression)
var foo = function Bar(){
return 7;
};
typeof Bar();
The output would be Reference Error. A function definition can have only one reference variable as its function name.
Q-CO2. What will be the output of the following code?
var y = 1;
if (function F(){}){
y += typeof F;
}
console.log(y);
The output would be undefined. The if condition statement evaluates using eval, so eval(function f(){}) returns function f(){} (which is true). Therefore, inside the if statement, executing typeof f returns undefined because the if statement code executes at run time, and the statement inside the if condition is evaluated during run time.
Q-CO3. What will be the output of the following code?
var output = (function(x){
delete X;
return X;
})(0);
console.log(output);
The output would be 0. The delete operator is used to delete properties from an object. Here x is not an object but a local variable. delete operators don’t affect local variables.
Q-CO4. What will be the output of the following code?
var x = { foo : 1};
var Output = (function() {
delete x.foo;
return x.foo;
})();
console.log(output);
The output would be undefined. An object’s property can be removed using the delete operator. In this case, object x holds the property foo. Since the function is self-invoking, we shall remove the foo property from object x. The outcome is still being determined when we attempt to reference a removed property after doing this.
Q-CO5. What will be the output of the following code?
var employee = {
company: 'xyz'
}
var emp1 = Object.create(employee);
delete Emp1.company;
console.log(emp1.company);
The output would be xyz. Here, emp1 object has company as its prototype property. The delete operator doesn’t delete prototype property. emp1 object doesn’t have company as its own property. However, we can delete the company property directly from the Employee object using delete employee.company.
Q-CO6. What will be the output of the following code?
// Code 1:
function func1(){
setTimeout(()=>{
console.log(x);
console.log(y);
},3000);
var x = 2;
let y = 12;
}
func1();
// Code 2:
function func2(){
for(var i = 0; i < 3; i++){
setTimeout(()=> console.log(i),2000);
}
}
func2();
// Code 3:
(function(){
setTimeout(()=> console.log(1),2000);
console.log(2);
setTimeout(()=> console.log(3),0);
console.log(4);
})();
Answers:
Code 1 - Outputs 2 and 12. Since, even though let variables are not hoisted, due to the async nature of JS, the complete function code runs before the setTimeout function. Therefore, it has access to both x and y.
Code 2 - Outputs 3, three times since variable declared with var keyword does not have block scope. Also, inside the for loop, the variable i is incremented first and then checked.
Code 3 - Output in the following order:
2
4
3
1 // After two seconds
Even though the second timeout function has a waiting time of zero seconds, the JS engine always evaluates the setTimeout function using the Web API, and therefore, the complete function executes before the setTimeout function can execute.
Q-CO7. What will be the output of the following code?
// Code 1:
let x= {}, y = {name:"Ronny"},z = {name:"John"};
x[y] = {name:"Vivek"};
x[z] = {name:"Akki"};
console.log(x[y]);
// Code 2:
function runFunc(){
console.log("1" + 1);
console.log("A" - 1);
console.log(2 + "-2" + "2");
console.log("Hello" - "World" + 78);
console.log("Hello"+ "78");
}
runFunc();
// Code 3:
let a = 0;
let b = false;
console.log((a == b));
console.log((a === b));
Answers:
Code 1 - Output will be {name: “Akki”}.
Adding objects as properties of another object should be done carefully.
Writing x[y] = {name:”Vivek”} , is same as writing x[‘object Object’] = {name:”Vivek”}.
While setting a property of an object, JS coerces the parameter into a string.
Therefore, since y is an object, it will be converted to ‘object Object’.
Both x[y] and x[z] are referencing the same property.
Code 2 - Outputs in the following order:
11
NaN
2-22
NaN
Hello78
Code 3 - Output in the following order due to equality coercion:
true
false
Q-CO8. What will be the output of the following code?
var x = 23;
(function(){
var x = 43;
(function random(){
x++;
console.log(x);
var x = 21;
})();
})();
Answer:
Output is NaN. The random() function has functional scope since x is declared and hoisted in the functional scope.
Rewriting the random function will give a better idea about the output:
function random(){
var x; // x is hoisted
x++; // x is not a number since it is not initialized yet
console.log(x); // Outputs NaN
x = 21; // Initialization of x
}
Q-CO9. What will be the output of the following code?
// Code 1
let hero = {
powerLevel: 99,
getPower(){
return this.powerLevel;
}
}
let getPower = hero.getPower;
let hero2 = {powerLevel:42};
console.log(getPower());
console.log(getPower.apply(hero2));
// Code 2
const a = function(){
console.log(this);
const b = {
func1: function(){
console.log(this);
}
}
const c = {
func2: ()=>{
console.log(this);
}
}
b.func1();
c.func2();
}
a();
// Code 3
const b = {
name:"Vivek",
f: function(){
var self = this;
console.log(this.name);
(function(){
console.log(this.name);
console.log(self.name);
})();
}
}
b.f();
Answers:
Code 1 - Output in the following order:
undefined
42
Reason - The first output is undefined since when the function is invoked, it is invoked referencing the global object:
window.getPower() = getPower();
Code 2 - Outputs in the following order:
global/window object
object "b"
global/window object
Since we are using the arrow function inside func2, this keyword refers to the global object.
Code 3 - Outputs in the following order:
"Vivek"
undefined
"Vivek"
Only in the IIFE inside the function f, this keyword refers to the global/window object.
Q-CO10. What will be the output of the following code?
Code 2 and 3 require you to modify the code, instead of guessing the output.
// Code 1
(function(a){
return (function(){
console.log(a);
a = 23;
})()
})(45);
// Code 2
// Each time bigFunc is called, an array of size 700 is being created,
// Modify the code so that we don't create the same array again and again
function bigFunc(element){
let newArray = new Array(700).fill('♥');
return newArray[element];
}
console.log(bigFunc(599)); // Array is created
console.log(bigFunc(670)); // Array is created again
// Code 3
// The following code outputs 2 and 2 after waiting for one second
// Modify the code to output 0 and 1 after one second.
function randomFunc(){
for(var i = 0; i < 2; i++){
setTimeout(()=> console.log(i),1000);
}
}
randomFunc();
Answers:
Code 1 - Outputs 45. Even though a is defined in the outer function, due to closure the inner functions have access to it.
Code 2 - This code can be modified by using closures,
function bigFunc(){
let newArray = new Array(700).fill('♥');
return (element) => newArray[element];
}
let getElement = bigFunc(); // Array is created only once
getElement(599);
getElement(670);
Code 3 - Can be modified in two ways:
Using let keyword:
function randomFunc(){
for(let i = 0; i < 2; i++){
setTimeout(()=> console.log(i),1000);
}
}
randomFunc();
Using closure:
function randomFunc(){
for(var i = 0; i < 2; i++){
(function(i){
setTimeout(()=>console.log(i),1000);
})(i);
}
}
randomFunc();
Q-CO11. Write the code for adding new elements dynamically?
<html>
<head>
<title>t1</title>
<script type="text/javascript">
function addNode(){
var newP = document. createElement("p");
var textNode = document.createTextNode("A new text node");
newP.appendChild(textNode);
document.getElementById("firstP").appendChild(newP);
}
</script>
</head>
<body>
<p id="firstP">firstP<p>
</body>
</html>
Q-CO12. In JavaScript, how do you turn an Object into an Array []?
let obj = { id: "1", name: "user22", age: "26", work: "programmer" };
//Method 1: Convert the keys to Array using - Object.keys()
console.log(Object.keys(obj));
// ["id", "name", "age", "work"]
// Method 2 Converts the Values to Array using - Object.values()
console.log(Object.values(obj));
// ["1", "user22r", "26", "programmer"]
// Method 3 Converts both keys and values using - Object.entries()
console.log(Object.entries(obj));
//[["id", "1"],["name", "user22"],["age", "26"],["work", “programmer"]]
Q-CO13. Write the code to find the vowels
const findVowels = str => {
let count = 0
const vowels = ['a', 'e', 'i', 'o', 'u']
for(let char of str.toLowerCase()) {
if(vowels.includes(char)) {
count++
}
}
return count
}
Q-CO14. What will be the output of the following code?
const b = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
for (let i = 0; i < 10; i++) {
setTimeout(() => console.log(b[i]), 1000);
}
for (var i = 0; i < 10; i++) {
setTimeout(() => console.log(b[i]), 1000);
}
Answer:
1
2
3
4
5
6
7
8
9
10
undefined
undefined
undefined
undefined
undefined
undefined
undefined
undefined
undefined
undefined
References:
Kommentare