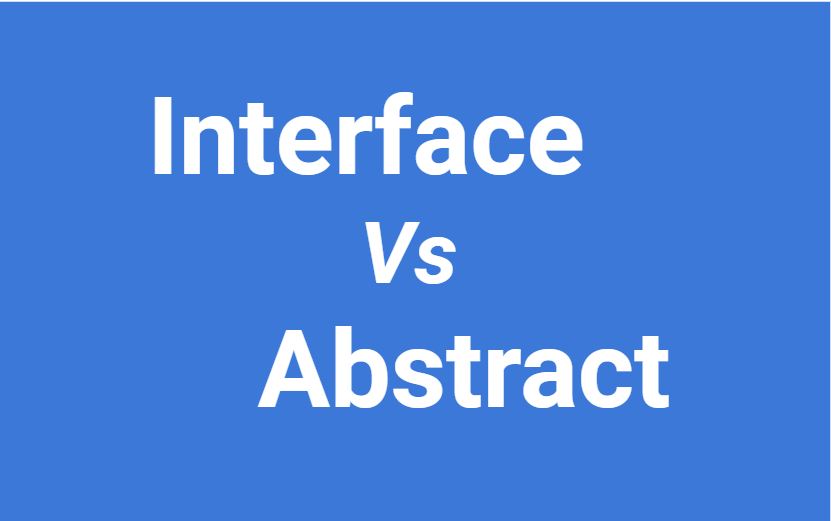
An immensely prevalent question used in interviewing. Let's have a quick look at what they are and how we can know which is abstract or interface.
Interface
The interface is a blueprint that can be used to implement a class. The interfaces:
Do not contain any concrete methods (methods that have code).
All the methods of an interface are abstract methods.
Cannot be instantiated. However, classes that implement interfaces can be instantiated.
Never contain instance variables but, they can contain public static final variables (i.e., constant class variables).
In short, An interface is an empty shell. There are only the signatures of the methods, which implies that the methods do not have a body. The interface can't do anything. It's just a pattern.
Abstract class
A class that has the abstract keyword in its declaration is called abstract class. Abstract classes:
Should have at least one abstract method. , i.e., methods without a body. It can have multiple concrete methods.
Allow you to create blueprints for concrete classes. But the inheriting class should implement the abstract method.
Cannot be instantiated.
In short, Abstract classes look a lot like interfaces, but they have something more: You can define behavior for them.
Code example
Interface Syntax:
Java Interface Sample:
Abstract Syntax:
Java Abstract Sample:
Comparisons
Methods:
Interface: can have only abstract methods. Since Java 8, it can have default and static methods also.
Abstract: can have abstract and non-abstract methods.
Variables:
Interface: has only static and final variables.
Abstract: can have final, non-final, static and non-static variables.
Access modifiers:
Interface: public by default.
Abstract: can have class members like private, protected, etc.
Extends:
Interface: an interface can extend another Java interface only.
Abstract: an abstract class can extend another Java class and implement multiple Java interfaces.
Implements:
Interface: cannot implement an abstract class.
Abstract: can implement an interface.
Inheritances:
Interface: an interface extending another interface is not responsible for implementing methods from the parent interface. This is because interfaces cannot define any implementation.
Abstract: when inheriting an abstract class, a concrete child class must define the abstract methods, whereas an abstract class can extend another abstract class and abstract methods from the parent class don't have to be defined.
A child class:
can extend only one abstract class but can implement many interfaces.
can define abstract methods with the same or less restrictive visibility, whereas a class implementing an interface must define the methods with the exact same visibility (public).
When to use?
An abstract class represents for "Is-A" relationship (Ex: Car is a MotorVehicle. Car must have all behaviors of MotorVehicle).
An interface represents for "Like-A" relationship (Ex: Car can Run. Run is an interface, Run is not a mandatory behavior of Car)
Make an abstract class when we want to supply directions for a specified class.
Make an interface when we want to supply some additional behaviors for a specified class and these behaviors are not mandatory for that class. Using interfaces is a substitution for multiple inheritances in Java.
Comments