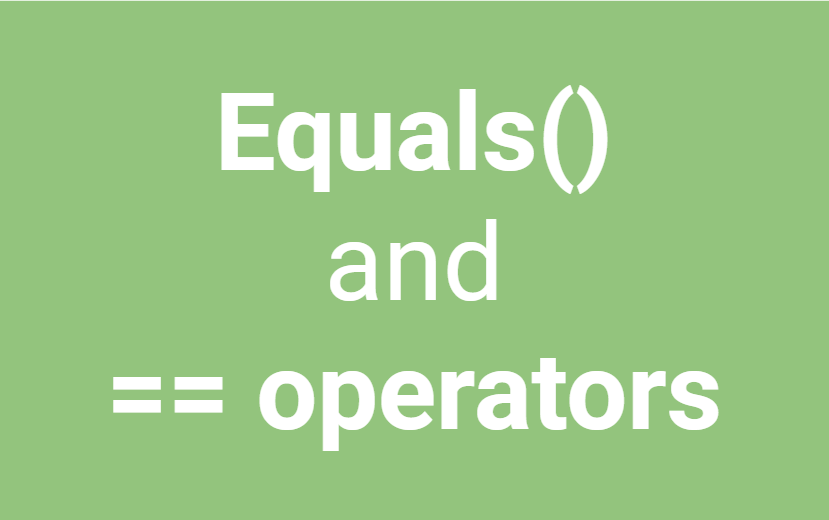
A prevalent and widely-used question in interviewing. In general, both equals() and “==” operator in Java are used to compare objects to check equality.
But they really have some of the differences between those two.
Differences:
Equals() is a method and == operator is a operator.
Equals() method for content comparison while == operators for reference comparison (address comparison). Long story short, == checks if both objects point to the same memory location whereas equals() evaluates to the comparison of values in the objects.
If a class does not override the equals() method, then by default, it uses equals(Object o) method of closest parent class that has overridden this method.
Code samples:
Output:
false
true
Explanation: We have created two String objects namely s1 and s2.
Both s1 and s2 refer to different objects.
When we use == operator for s1 and s2 comparison then the result is false since both have different addresses in memory.
Using equals(), the result is true because it's only comparing the actual values given in s1 and s2.
Now let go in detail of both in Java.
Equality Operator ==
Definition:
"==" or equality operator in Java used to compare primitives and objects.
In terms of comparing primitives like boolean, int, float "==" works fine but when it comes to comparing objects it creates confusion with equals method in Java.
"==" compare two objects based on memory reference. so "==" operator will return true only if two object references it is comparing represent exactly the same object otherwise "==" will return false.
Equals() method
Definition:
Equals() method is defined in Object class and used for checking equality of two objects defined by business logic
Ex: two Employees are considered equal if they have the same empId etc.
We can have your domain object and then override equals() method for defining a condition on which two domain objects will be considered equal.
Compare Strings with == operator and equals()
String comparison is a common scenario of using both == and equals method.
since java String class overrides equals() method, It returns true if two String object contains the same content.
but == will only return true if two references are pointing to the same object.
Here is an example of comparing two Strings in Java for equality using == and equals() method which will clear some doubts:
Output: Comparing two strings with == operator: false Comparing two Strings with the same content using equals method: true Comparing two references pointing to same String with == operator: true
Compare Objects with == operator and equals()
Another scenario that creates confusion between == and equals method is when you compare two Objects. When you compare two references pointing to an object of type Object you should see the same result from both == operator and equals method because the default implementation of equals method just compares memory address of two objects and returns true if two reference variables are pointing towards an exactly same object. Here is an example of == vs equals method for comparing two objects:
Output: Comparing two different Objects with == operator: false Comparing two different Objects with equals() method: false Comparing two references pointing to the same Object with == operator: true
Summary:
Use == to compare primitive e.g. boolean, int, char etc, while use equals() to compare objects in Java.
== return true if two references are of the same object. The result of equals() method depends on overridden implementation.
For comparing String use equals() instead of == equality operator.
Comments